Blog
All Blog Posts | Next Post | Previous Post
Generate QR codes with TMS WEB Core
Tuesday, August 4, 2020
TMS WEB Core already has a TWebQRDecoder component to decode QR codes. It's evident that we need something that works the other way around and creates QR codes instead. Meet our new addition to tackle this problem: TWebQRCode.TWebQRCode is a wrapper around the qrcode.js JavaScript library. qrcode.js is a small library with the purpose of creating a QR code based on a string input.
Functionality
Not surprisingly, you can generate a QR code based on a text. Just drop the component onto the form, and set the TWebQRCode.Text property:procedure TForm1.WebButton1Click(Sender: TObject); begin WebQRCode1.Text := WebEdit1.Text; end;
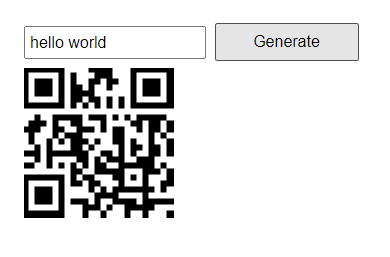
You can also retrieve the QR code as a base64 encoded string or as a TBitmap. To retrieve this data, use the GetBase64/GetBitmap methods or their GetBase64Async/GetBitmapAsync versions. The reason behind an async version of these methods is simple: GetBase64 and GetBitmap will work right away if the component is visible, because the underlying image source is a base64 encoded string. If you want to get a QR code data without showing any images, then the process will be asynchronous because the image needs to be created and loaded on the fly by the library. These asynchronous versions work with anonymous methods. This way you have full control over the retrieved data.
procedure TForm1.WebButton1Click(Sender: TObject); var qr: TWebQRCode; begin qr := TWebQRCode.Create(Self); qr.Width := 200; qr.Height := 200; qr.Text := 'my text'; qr.GetBase64Async(procedure (AData: string) begin //do something with AData end); end;
procedure TForm1.WebButton1Click(Sender: TObject); begin WebQRCode1.Download('myqrcode.png'); end;
Example
As a small example, we are going to create a small business card generator application with TTMSFNCPDFLib. TTMSFNCPDFLib makes it possible to create PDF files on the fly and if we combine it with TWebQRCode then we have an application which can generate PDF files with QR codes directly in the client!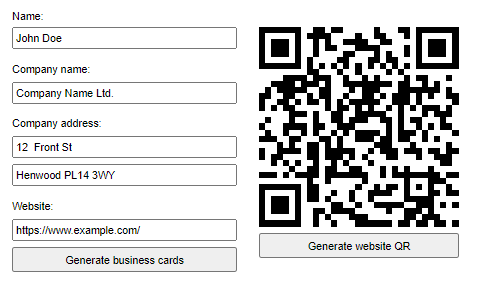
To keep it short, we are going to focus on the QR code drawing. As a first step, we need to load the default fonts and disable the button until the fonts are loaded.
procedure TForm1.WebFormCreate(Sender: TObject); begin WebButton2.Enabled := False; Application.OnFontCacheReady := DoFontCacheReady; AddFontToCache('https://download.tmssoftware.com/tmsweb/pdf/fonts/Roboto-Regular.ttf'); end; procedure TForm1.DoFontCacheReady(Sender: TObject); begin WebButton2.Enabled := True; end;
procedure TForm1.WebButton2Click(Sender: TObject); var PDF: TTMSFNCPDFLib; I: Integer; begin WebQRCode1.Text := edtWebsite.Text; //Set URL WebQRCode1.GetBitmapAsync(procedure (ABitmap: TBitmap) //Wait for bitmap to load begin PDF := TTMSFNCPDFLib.Create; try PDF.BeginDocument('sample.pdf'); PDF.PageSize := psA4; PDF.Header := ''; PDF.Footer := ''; PDF.NewPage; PDF.Graphics.Font.Name := 'Roboto'; PDF.Graphics.Font.Color := gcBlack; PDF.Graphics.Font.Style := []; //Draw with PDF here PDF.EndDocument(True); finally PDF.Free; end; end); end;
procedure TForm1.DrawBusinessCard(APDF: TTMSFNCPDFLib; ALeft, ATop, ARight, ABottom: Integer; AQR: TBitmap); begin APDF.Graphics.DrawRectangle(RectF(ALeft, ATop, ARight, ABottom)); //text drawing would come here APDF.Graphics.DrawImage(AQR, RectF(ARight - 95, ABottom - 90, ARight - 25, ABottom - 20)); end;
But wait, we have some more good news: we made this component available through our TMS WEB Core Partner program which means that you can download it from here for free!
To install, open, compile & install the package from the "Component Library Source" folder. This will install the design-time TWebQRCode component.
For use at runtime, make sure that the "Core Source" folder is in your TMS WEB Core specific library path that you can set via IDE Tools, Options, TMS Web, Library path.
Tunde Keller

This blog post has received 5 comments.


https://www.tmssoftware.com/site/webpartners.asp
Bruno Fierens

van Heuven Rob


https://www.tmssoftware.com/site/webpartners.asp
Bruno Fierens

User
All Blog Posts | Next Post | Previous Post
Patrick