Blog
All Blog Posts | Next Post | Previous Post
Small XLSX component to deal with Excel files in TMS Web Core applications
Tuesday, August 25, 2020
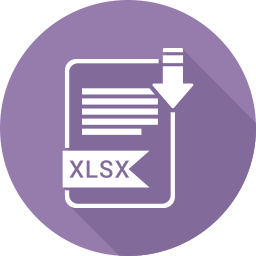
Many times a quick and easy way is needed to export some basic data from a grid into an Excel file. We all know that Excel has lots of various features and covering the offered functionalities would require a huge library that we probably don't need for smaller tasks. We wanted to create something for these smaller tasks that covers reading, editing and writing Excel files locally in the client application while avoiding the complexities of a huge library.
TWebXLSX
TWebXLSX is a new free non-visual component as a wrapper around the open-source ExcelJS JavaScript library. Keep in mind, that ExcelJS only supports XLSX format.Loading an excel workbook is as easy as calling TWebXLSX.Load with an array buffer as a parameter. In case of a TWebFilePicker, loading an XLSX file looks like this:
procedure TForm1.WebFilePicker1Change(Sender: TObject); begin if WebFilePicker1.Files.Count > 0 then WebFilePicker1.Files.Items[0].GetFileAsArrayBuffer; end; procedure TForm1.WebFilePicker1GetFileAsArrayBuffer(Sender: TObject; AFileIndex: Integer; ABuffer: TJSArrayBufferRecord); begin WebXLSX1.Load(ABuffer); end;
ExcelJS is a workbook manager and it does not provide a visual element to display the data that it contains. Because of this, we added a Grid property where you can assign a TWebStringGrid or a TWebTableControl depending on your needs. TWebXLSX will load the data from the first sheet of the workbook into the grid automatically. If you want to change between the different sheets, you can use the TWebXLSX.ActiveSheet string property to define the active sheet name. If you don't know what sheet names are available, you can loop through the TWebXLSX.SheetNames property to find them out.
procedure TForm1.WebXLSX1WorkbookLoaded(Sender: TObject); var I: Integer; begin for I := 0 to WebXLSX1.SheetNameCount - 1 do WebListBox1.Items.Add(WebXLSX1.SheetNames[I]); end;
Sometimes a workbook contains sheets that don't have any data. You can detect if a sheet has any rows by calling TWebXLSX.IsEmptySheet('sheetname').
When it comes to saving a workbook, you might want to apply some basic cell formatting. For this you can implement the OnSaveCell event which is triggered for each cell when the active sheet is saved (e.g. changing sheets or saving the workbook). With this event, you have direct access to the underlying ExcelJS cell object. Check out the styling documentation of ExcelJS to see which style settings are supported. You can also check the core libexceljs.pas file to see which properties we mapped already. If something that you'd like to use is missing, you can always extend it yourself!
procedure TForm1.WebXLSX1SaveCell(Sender: TObject; ARow, AColumn: Integer; AContent: string; var ACell: TJSExcelJSCellRecord); begin if ARow = 0 then ACell.cell.style.alignment.horizontal := 'center'; end;
And last but not least, you can use the OnWorkbookLoaded event when a workbook has finished loading, the OnSheetLoaded event when a sheet has finished loading into the grid and the OnNewSheetAdded event when a new sheet is added to the workbook.
Are you interested to see the TWebXLSX component in action? Check out our demo by clicking the button below!
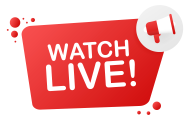
As mentioned at the beginning of the artice, TWebXLSX is a free component that we are releasing as part of our TMS WEB Core Partner program and you can download it from here!
To install, open, compile & install the package from the "Component Library Source" folder. This will install the design-time TWebXLSX component.
For use at runtime, make sure that the "Core Source" folder is in your TMS WEB Core specific library path that you can set via IDE Tools, Options, TMS Web, Library path.
Tunde Keller

This blog post has received 9 comments.

Wynne Mark


Please contact technical support with exact details.
Bruno Fierens

But do I understand this right, it is only intended to use on Windows, not on Linux or MacOS ( I can not add an other platform to the prject)?
Harry Stahl


Bruno Fierens

The component don''t work on Delphi 11 Alexandria.
Please can you provide an update?
On my system we updated TMSWEBCorePkgLibDXE13 to TMSWEBCorePkgLibDXE14 but when we try to install it raises an access violation error.
Dor Bujor Padureanu


Opened ExcelJSXLSX.dpk, changed reference in requires to TMSWEBCorePkgLibDXE14 and then compiled & installed this package without any issue.
Bruno Fierens

* [dcc32 Error] ExcelJSXLSX.dpk(36): E2200 Package ''TMSWEBCorePkgLibDXE12'' already contains unit ''WEBLib.XLSX''
* [dcc32 Error] ExcelJSXLSX.dpk(37): E2200 Package ''TMSWEBCorePkgLibDXE12'' already contains unit ''libexceljs''
When I go exploring, I find a very different WEBLib.XLSX.pas in Web Core\Core Source. Can you tell me what I need to do to get ExcelJS to work in Web Core now?
Berends Richard


Bruno Fierens
All Blog Posts | Next Post | Previous Post
Hennekens Stephan