TAdvStringGrid
Example 84 : Customizing the item class in TAdvGridDropDown
TAdvGridDropDown can hold the information shown in the dropdown grid in the Items collection. The component TAdvGridDropDown was designed to offer a customizable item collection. With a customizable item collection, additional properties can be added that control for example appearance or behavior of cells in the grid dropdown.TAdvGridDropDown has by default a collection of items of the type TDropDownItems. The item class in the TDropDownItems collection is TDropDownItem. By creating a new class TAdvGridDropDownEx, descending from TAdvGridDropDown, the virtual method CreateItems can be overridden to create a custom collection. At the same time, the override of the GetItemClass function in the items collection class can be used to return a new class, descending from TDropDownItem. Summarized, in code this looks like:
TDropDownItemEx = class(TDropDownItem) end; TDropDownItemsEx = class(TDropDownItems) protected function GetItemClass: TDropDownItemClass; override; end; TAdvGridDropDownEx = class(TAdvGridDropDown) protected function CreateItems(AOwner: TComponent): TDropDownItems; override; end;
In this sample, we apply this technique to create a TDropDownItem descending class where a Color and FontStyle property is added. This Color and FontStyle will be used to control the appearance of the items in the dropdown grid.
Adding the Color and FontStyle property in TDropDownItemEx is done with:
TDropDownItemEx = class(TDropDownItem) private FTag: integer; FColor: TColor; FFontStyle: TFontStyles; public constructor Create(Collection: TCollection); published property Color: TColor read FColor write FColor; property FontStyle: TFontStyles read FFontStyle write FFontStyle; property Tag: integer read FTag write FTag; end; { TDropDownItemEx } constructor TDropDownItemEx.Create(Collection: TCollection); begin inherited; FTag := 0; FColor := clWindow; FFontStyle := []; end;
Then, in TAdvGridDropDownEx, the virtual method DoGridGetCellColor is overridden to use the extra information in the item class to set a background color and font style:
TAdvGridDropDownEx = class(TAdvGridDropDown) protected procedure DoGridGetCellColor(Sender: TObject; ARow, ACol: Integer; AState: TGridDrawState; ABrush: TBrush; AFont: TFont); override; function CreateItems(AOwner: TComponent): TDropDownItems; override; end; procedure TAdvGridDropDownEx.DoGridGetCellColor(Sender: TObject; ARow, ACol: Integer; AState: TGridDrawState; ABrush: TBrush; AFont: TFont); begin inherited; if (ARow >= Grid.FixedRows) then begin ABrush.Color := (Items[ARow - Grid.FixedRows] as TDropDownItemEx).Color; AFont.Style := (Items[ARow - Grid.FixedRows] as TDropDownItemEx).FontStyle; end; end;
From application code, the items are initialized with the code:
begin AdvGridDropDownEx1.Items.Clear; with (AdvGridDropDownEx1.Items.Add as TDropDownItemEx) do begin Color := clInfoBk; FontStyle := [fsBold]; Text.Add('Row 1 C1'); Text.Add('Row 1 C2'); Text.Add('Row 1 C3'); end; with (AdvGridDropDownEx1.Items.Add as TDropDownItemEx) do begin Color := clWhite; FontStyle := [fsItalic]; Text.Add('Row 2 C1'); Text.Add('Row 2 C2'); Text.Add('Row 2 C3'); end; with (AdvGridDropDownEx1.Items.Add as TDropDownItemEx) do begin Color := clYellow; FontStyle := []; Text.Add('Row 3 C1'); Text.Add('Row 3 C2'); Text.Add('Row 3 C3'); end; with (AdvGridDropDownEx1.Items.Add as TDropDownItemEx) do begin Color := clInfoBk; FontStyle := [fsUnderline]; Text.Add('Row 4 C1'); Text.Add('Row 4 C2'); Text.Add('Row 4 C3'); end; end;
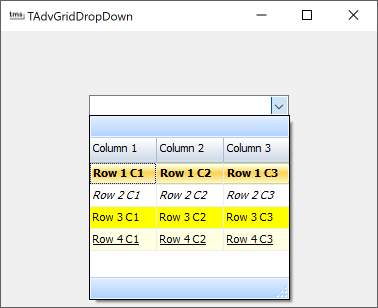
×