TAdvStringGrid
Example 7 in C++Builder : Setting cell color, alignment & sorting styles
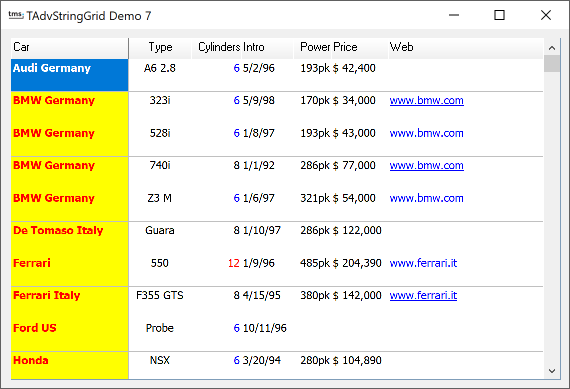
First of all, to populate the grid, data from a CSV file is used. In the FormCreate routine, this data is loaded. The column header text is set at design time, using the ColumnHeaders property. This is the code to load the data :
void __fastcall TForm1::FormCreate(TObject *Sender) { AdvStringGrid1->SaveFixedCells=FALSE; AdvStringGrid1->LoadFromCSV("sample.csv"); AdvStringGrid1->AutoSizeColumns(FALSE,8); }
Next, the OnGetCellColor event is used to set the cell color & font properties for each cell. This event handler is called for every cell and allows to choose different fonts, colors and font styles for each cell. In this example, only the color for the first column is changed and the color of the "Cylinder" column is changed depending on the value of the cell.
void __fastcall TForm1::AdvStringGrid1GetCellColor(TObject *Sender, long ARow, long ACol, TGridDrawState AState, TBrush *ABrush, TFont *AFont) { if ((ACol==0) && (ARow>0)) { ABrush->Color = clYellow; AFont->Color = clRed; AFont->Style << fsBold; } if ((ACol==2) && (ARow>0)) { if (AdvStringGrid1->Cells[ACol][ARow]=="4") AFont->Color=clGreen; if (AdvStringGrid1->Cells[ACol][ARow]=="6") AFont->Color=clBlue; if (AdvStringGrid1->Cells[ACol][ARow]=="8") AFont->Color=clBlack; if (AdvStringGrid1->Cells[ACol][ARow]=="12") AFont->Color=clRed; } }
void __fastcall TForm1::AdvStringGrid1GetAlignment(TObject *Sender, long ARow, long ACol, TAlignment &AAlignment) switch(ACol) { case 1: AAlignment=taCenter; break; case 2,4: AAlignment=taRightJustify; break; } }
TSortStyle = (ssAutomatic, ssAlphabetic, ssNumeric, ssDate, ssAlphaNoCase, ssAlphaCase, ssShortDateEU, ssShortDateUS, ssCustom, ssFinancial); void __fastcall TForm1::AdvStringGrid1GetFormat(TObject *Sender, long ACol, TSortStyle &AStyle, AnsiString &aPrefix, AnsiString &aSuffix) { switch(ACol) { case 0: AStyle=ssAlphaNoCase; break; case 1: AStyle=ssAlphaNoCase; break; case 2: AStyle=ssNumeric; break; case 3: AStyle=ssDate; break; case 4: AStyle=ssNumeric; aSuffix="pk"; break; case 5: AStyle=ssNumeric; aPrefix="$"; break; } }
As a last extension, the hinting of the grid is changed for each column. :
void __fastcall TForm1::AdvStringGrid1GridHint(TObject *Sender, long Arow, long Acol, AnsiString &hintstr) { switch(Acol) { case 0: hintstr="Car manufacturer"; break; case 1: hintstr="Car model"; break; case 2: hintstr="Nr. of cylinders in model"; break; case 3: hintstr="Introduction date"; break; case 4: hintstr="Engine horse power"; break; case 5: hintstr="Date of production start"; break; case 6: hintstr="Link to manufacturer website"; break; } }
×